Hi everyone, I want to share you about how to create if conditional inside a map() function in React.
When you develop React application, sometimes you need to display item base on a condition especially in map().
However, when you using map()
function in JavaScript/TypeScript is crafty.
But, no worries. I will explain to you how to create conditional to display data in looping map().
# Before Begin
For the first time, I have initialize React project using Vite and the structure following like this below.
The setup generator using Vite would create some files like App.css, App.tsx, index.css, main.tsx, etc.
Feel free to use others setup like using Next.js or online react app editor like CodeSandbox, etc.
This following structure that I created before:
-- src
|-- App.css
|-- App.tsx
|-- index.css
|-- main.tsx
App.css
file
#root {
max-width: 1280px;
margin: 0 auto;
padding: 2rem;
text-align: center;
}
App.tsx
file
import './App.css';
function App() {
return (
<>
<p>Hello React</p>
</>
)
}
export default App;
index.css
file
:root {
font-family: Inter, system-ui, Avenir, Helvetica, Arial, sans-serif;
line-height: 1.5;
font-weight: 400;
color: rgba(255, 255, 255, 0.87);
background-color: #242424;
}
main.tsx
file
import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App.tsx';
import './index.css';
ReactDOM.createRoot(document.getElementById('root')!).render(
<React.StrictMode>
<App />
</React.StrictMode>,
)
# Example of React App
In this article, generally I will create react application that showing list of the countries with GDP per capita (in USD).
I this case, I have edit files to show data from five countries like this following code:
File App.tsx
import './App.css';
interface ICountry {
id: number;
name: string;
GDPCapita: number;
}
function App() {
const listOfCountries: ICountry[] = [
{ id: 1, name: "Australia", GDPCapita: 65099 },
{ id: 2, name: "Algeria", GDPCapita: 4342 },
{ id: 3, name: "Japan", GDPCapita: 33823 },
{ id: 4, name: "Germany", GDPCapita: 48717 },
{ id: 5, name: "India", GDPCapita: 2410 },
];
return (
<>
<h3>List Of Countries</h3>
{listOfCountries.map((country: ICountry) => (
<div key={country?.id} className="container">
<p><strong>{country?.name}</strong></p>
<p>GDP/capita: {country?.GDPCapita} USD</p>
</div>
))}
</>
)
}
export default App;
In the file App.tsx
, I want to show list of card that showing list from five countries from Australia, Algeria, Japan, Germany, and India.
Furthermore, I would to define the data as listOfCountries
variable that contains data such as id
, name
, and also GPD per capita from the country as GDPCapita
.
I also using interface to define structure from country object as ICountry
.
File App.css
#root {
max-width: 1280px;
margin: 0 auto;
padding: 1rem;
text-align: center;
}
.container {
border: 1px solid #ccc;
max-width: 300px;
margin: 0 auto;
margin-bottom: 5px;
}
For styling, I only added new .container
class for make card more visible in the App.css
file.
Output
As a result, after changed the React code then user interface will be output like this.
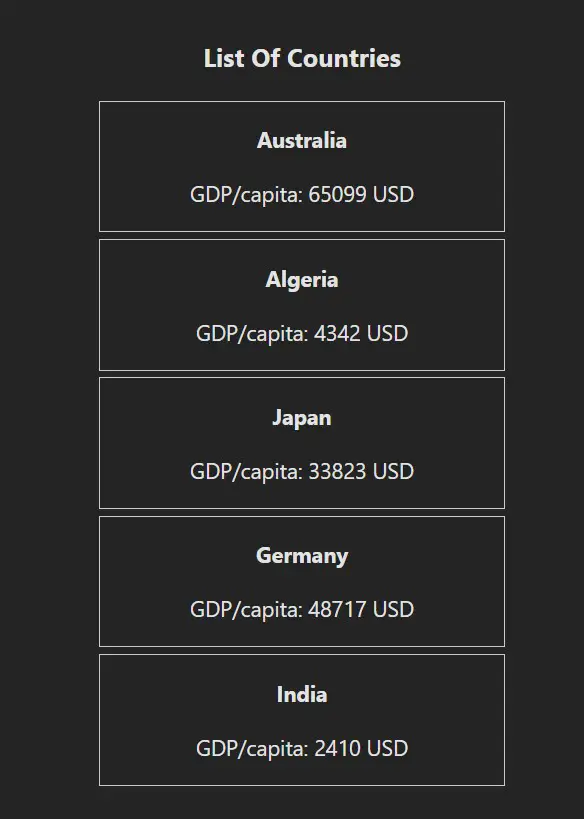
I think that is what was expected.
Let’s say we will show only country with GDP per capita more than 5.000 USD.
The problem is how to filtering data (in this case) list of the countries that has GPD per capita more than 5.000 USD per year.
Using map() looping method is very tricky.
However, we can handle this condition using many different approaches.
Let’s begin.
# Solution 1: if Condition
First, you can use if statement inside map() and return null when GPD per capita less than USD 5,000.
import './App.css';
interface ICountry {
id: number;
name: string;
GDPCapita: number;
}
function App() {
const listOfCountries: ICountry[] = [
{ id: 1, name: "Australia", GDPCapita: 65099 },
{ id: 2, name: "Algeria", GDPCapita: 4342 },
{ id: 3, name: "Japan", GDPCapita: 33823 },
{ id: 4, name: "Germany", GDPCapita: 48717 },
{ id: 5, name: "India", GDPCapita: 2410 },
];
return (
<>
<h3>List Of Countries</h3>
{listOfCountries.map((country: ICountry) => {
if (country?.GDPCapita < 5000) return null;
return (
<div key={country?.id} className="container">
<p><strong>{country?.name}</strong></p>
<p>GDP/capita: {country?.GDPCapita} USD</p>
</div>
)
})}
</>
)
}
export default App;
# Solution 2: Using ternary operator condition
Secondly, you can use ternary operator inside map() method using syntax:
condition ? <if true> : <if false>
So, if GPD per capita more than USD 5,000 that will showing the data. I edit into this following code:
...
function App() {
const listOfCountries: ICountry[] = [
...
];
return (
<>
<h3>List Of Countries</h3>
{listOfCountries.map((country: ICountry) => (
country?.GDPCapita > 5000 ? (
<div key={country?.id} className="container">
<p><strong>{country?.name}</strong></p>
<p>GDP/capita: {country?.GDPCapita} USD</p>
</div>
) : null
))}
</>
)
}
export default App;
# Solution 3: Using Logical (AND)
We also can using logical && to showing country with GDP per capita more than USD 5,000.
...
function App() {
const listOfCountries: ICountry[] = [
...
];
return (
<>
<h3>List Of Countries</h3>
{listOfCountries.map((country: ICountry) => (
<>
{country?.GDPCapita > 5000 && (
<div key={country?.id} className="container">
<p><strong>{country?.name}</strong></p>
<p>GDP/capita: {country?.GDPCapita} USD</p>
</div>
)}
{country?.GDPCapita <= 5000 && null}
</>
))}
</>
)
}
export default App;
Output
Therefore, after filtering data that only GDP per capita more than USD 5,000 the result is like below.
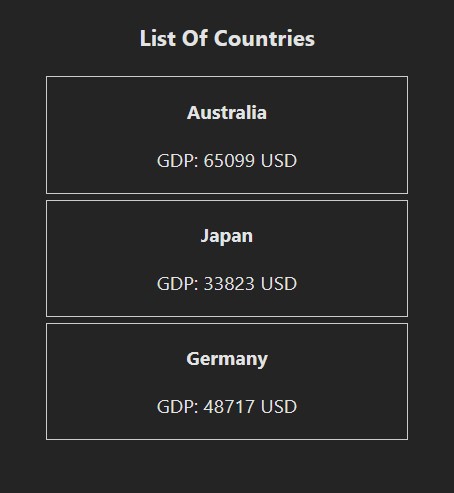
# Conclusion
If you have any questions or suggestion, please write in the comment below.
Or do you have any other ideas to create condition inside map in React, please share it.
I think there are many solution to adding if condition inside the map method in React.
Also, please check my previous article about add onClick on React here.
Thanks. Happy coding.