Hello everybody,
Today, I will show you how to add onClick Event in ReactJS library.
Before that, I think you are familiar with React for developing web application.
# Project Setup
For now, I am using Vite to initialize react project.
For alternative, you can using options like Next.js, Remix, CodeSanbox, and many more.
If you using online code editor like CodeSanbox, you can skip this step to init react project.
To initialize project you can following this command from terminal or CMD.
$ npm create vite@latest
After you run the command above, the result will showing below:
Ok to proceed? (y) y
√ Project name: … your-application-name
√ Select a framework: » React
√ Select a variant: » JavaScript
It is my React file structure directories
--- src
------ App.css
------ App.jsx
------ index.css
------ main.jsx
Then, to running React application you can go to directory app, install packages using npm install
, and run React app using npm run dev
.
$ cd your-application-name
$ npm install
$ npm run dev
After running app, you can check on the browser on `http://localhost:5173/`. Maybe, this have different port base on your app.
Next, we will focus on main file on the src/App.jsx
It is my App.js
file
import './App.css'
function App() {
return (
<h1>React App</h1>
)
}
export default App
And it is my App.css
file only for styling.
#root {
max-width: 1280px;
margin: 0 auto;
padding: 2rem;
text-align: center;
}
# Create onClick React event directly
This following onClick method can directly put on button
tag.
So, when you click the button, it will be triggered.
Not only button, you can click another tag like div, span, etc.
onClick={() => todo here .... }
import './App.css'
function App() {
return (
<>
<h1>React onClick App</h1>
<button onClick={() => console.log("Hello React.js")}>Click Me!</button>
</>
)
}
export default App;
Result:
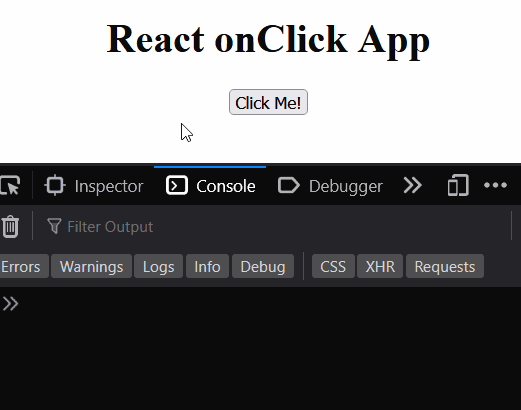
# Create onClick React as a function
You can create a method/function to trigger onClick event.
If method clicked, you can do something like send data or do anything here.
const handleButtonClick = () => {
…. todo here …. }
import './App.css'
function App() {
const handleButtonClick = () => {
console.log('Your button clicked!');
}
return (
<>
<h1>React onClick App</h1>
<button onClick={handleButtonClick}>Click Me!</button>
</>
)
}
export default App;
Result:
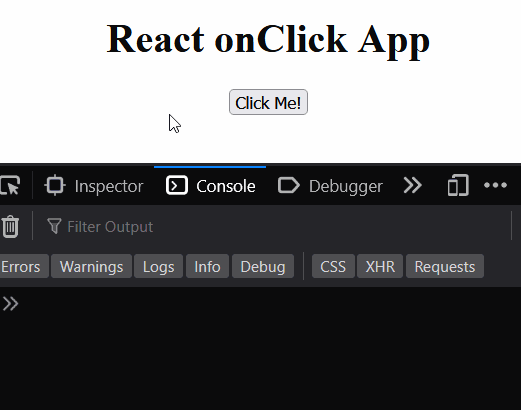
# Create onClick React to pass parameter
As we know that, you can pass argument into function.
If you want to get argument value, React can handling it.
You can show value from parameter.
For example, this following code, I send argument John Doe
as a parameter name
and you will get result Hello John Doe
from console.
import './App.css'
function App() {
const handleButtonClick = (name) => {
console.log(`Hello ${name}`);
}
return (
<>
<h1>React onClick App</h1>
<button onClick={() => handleButtonClick('John Doe')}>Click Me!</button>
</>
)
}
export default App;
Result:
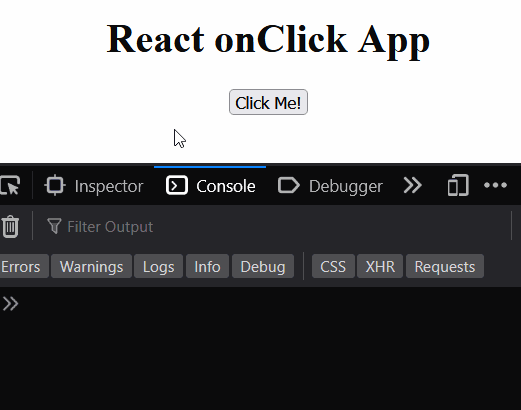
# Create onClick for set state in React
You can use onClick to set state in React.js.
This following code is example using useState hook.
If state from show
is false
, the name is not show.
Otherwise, when you click button, the state data show
will be changed into true
.
Then, the result name will be showing.
import './App.css';
import { useState } from 'react';
function App() {
const [show, setShow] = useState(false);
return (
<>
<h1>React onClick App</h1>
<button onClick={() => setShow(true)}>Click Here!</button>
{ show && <p>My name is John Doe</p> }
</>
)
}
export default App;
Result:
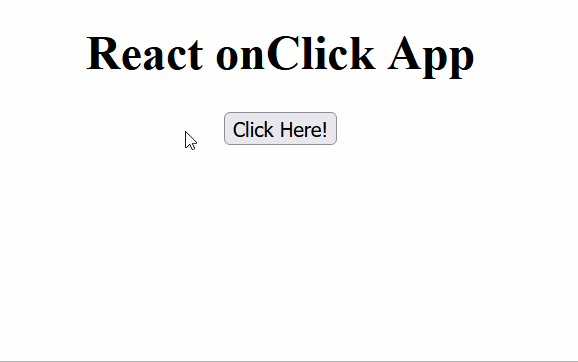
# Conclusion
Event onClick in React Js is very interesting to learn it.
You can use this event in your web application in any method.
Happy coding! 🙂