Hello everyone, In this article I want to share you about how to save or add data on the localStorage
using React JS.
Do you know what is localStorage in the browser?
The primary purpose from localStorage is enable programmer to storing data and retrieve some important data in the browser.
With localStorage
, you can use to save login information, set dark theme, save item, and etc.
In this article, we will try to use localStorage to save item, get item, and to remove item.
# Project Setup
First of all, you must install react project in your local computer or using online tools like CodeSandbox.
I choose Vite to initialize my react project.
$ npm create vite@latest
For init project, this is my structure files for React application.
src
|----- App.css
|----- App.jsx
|----- main.jsx
This is my files,
App.css
#root {
max-width: 1280px;
margin: 0 auto;
padding: 2rem;
text-align: center;
}
main.jsx
import React from 'react'
import ReactDOM from 'react-dom/client'
import App from './App.jsx'
import './index.css'
ReactDOM.createRoot(document.getElementById('root')).render(
<React.StrictMode>
<App />
</React.StrictMode>,
)
App.jsx
import './App.css';
function App() {
return (
<h1>React App</h1>
)
}
export default App;
# Save localStorage Using onClick Event
We can store localStorage using method setItem in JavaScript browser.
For example, this following code when I click the button will trigger event to save into the local storage.
I use setItem
method to save data ‘name’ with value ‘John’ into localStorage.
import './App.css';
function App() {
const handleSaveData = () => {
window.localStorage.setItem('name', 'John');
}
return (
<>
<h1>React App</h1>
<p>Save data into localStorage</p>
<button onClick={handleSaveData}>Save</button>
</>
)
}
export default App;
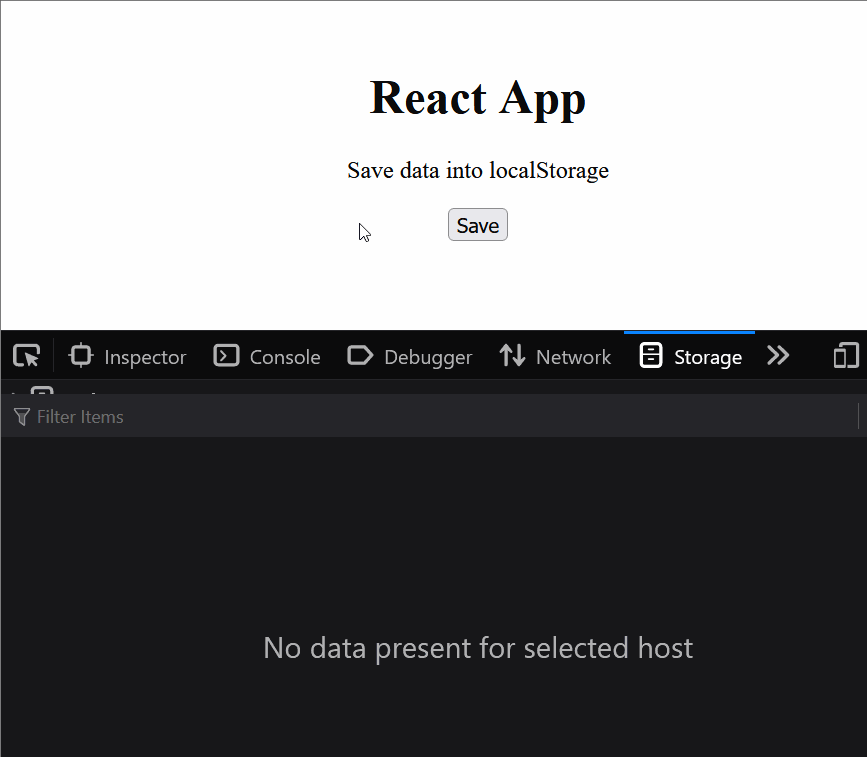
# Retrive and Remove Data From localStorage
As we know, you can retrieve data from localStorage using method localStorage.getItem('...')
.
In the other hand, you can remove localStorage item using method removeItem(value, ...)
import './App.css';
function App() {
const handleSaveData = () => {
window.localStorage.setItem('name', 'John');
}
const handleRemoveData = () => {
const dataItem = window.localStorage.getItem('name');
if (dataItem) {
window.localStorage.removeItem('name');
} else {
alert('sorry, your data item is not found.');
}
}
return (
<>
<h1>React App</h1>
<p>Save data into localStorage</p>
<button onClick={handleSaveData}>Save</button>
<button onClick={handleRemoveData}>Remove</button>
</>
)
}
export default App;
For example, from the code above you can store data when click ‘Save’ button. Then, name ‘John’ will be saved into local storage.
Then, when you click ‘Remove’ button the browser will checking the name data from localStorage.
If ‘name’ exist then data ‘name’ will removed from localStorage. And, when not exist the browser will show the alert.
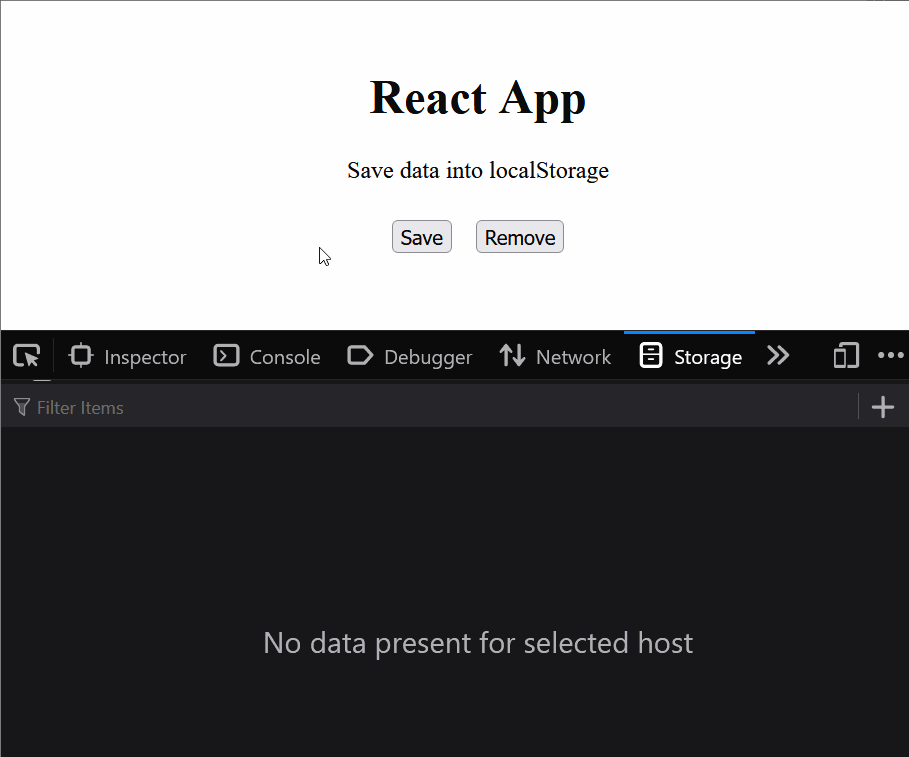
# Conclusion
Okay, in this article we learn a lot about how to add data on the localStorage
in React.js application.
So, do you want to try this?
Thank you. Happy coding!